Understanding the `preventDefault()` Function in JavaScript
by Codrut Radu, Senior Developer
JavaScript is a versatile programming language that powers much of the interactivity we experience on the web today. When building web applications, developers often encounter scenarios where they need to control how a web page behaves in response to user interactions, such as clicking a link or submitting a form. One powerful tool in the JavaScript toolbox for handling these interactions is the preventDefault()
function. This article will explore what preventDefault()
does, when and why it is used, and provide code examples to illustrate its practical applications.
1. What is preventDefault()
?
The preventDefault()
function is a method that belongs to the Event
interface in JavaScript. It is primarily used to stop an event's default behavior from occurring. Events can include clicking on links, submitting forms, pressing keys, and more. These default behaviors can sometimes interfere with the desired functionality of a web application, and that's where preventDefault()
comes into play.
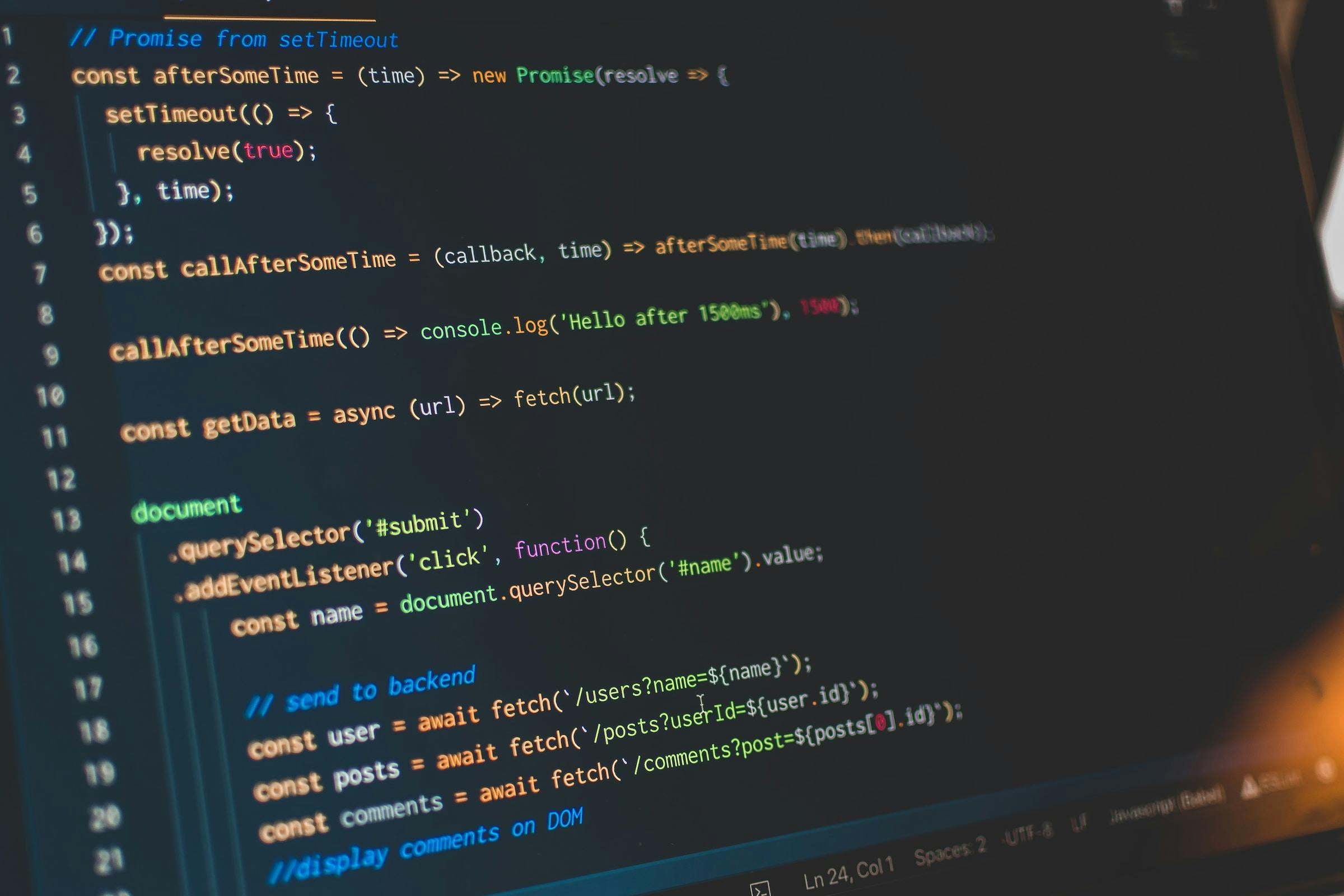
2. When is preventDefault()
Used?
preventDefault()
is typically used when you want to customize the behavior of an event.
For example:
- Link Clicks: When a user clicks on a link (
<a>
element), the default behavior is to navigate to the URL specified in the href attribute. If you want to intercept and handle the click event using JavaScript without navigating away from the current page, you can usepreventDefault()
. - Form Submissions: When a user submits a form (
<form>
element), the default behavior is to send data to the server and reload the page. If you want to validate user input with JavaScript before submitting the form or handle the form submission via AJAX, you can usepreventDefault()
to prevent the default submission. - Key Presses: In cases where you want to capture key presses and perform custom actions (e.g., keyboard shortcuts), you can use
preventDefault()
to prevent the default behavior associated with specific keys.
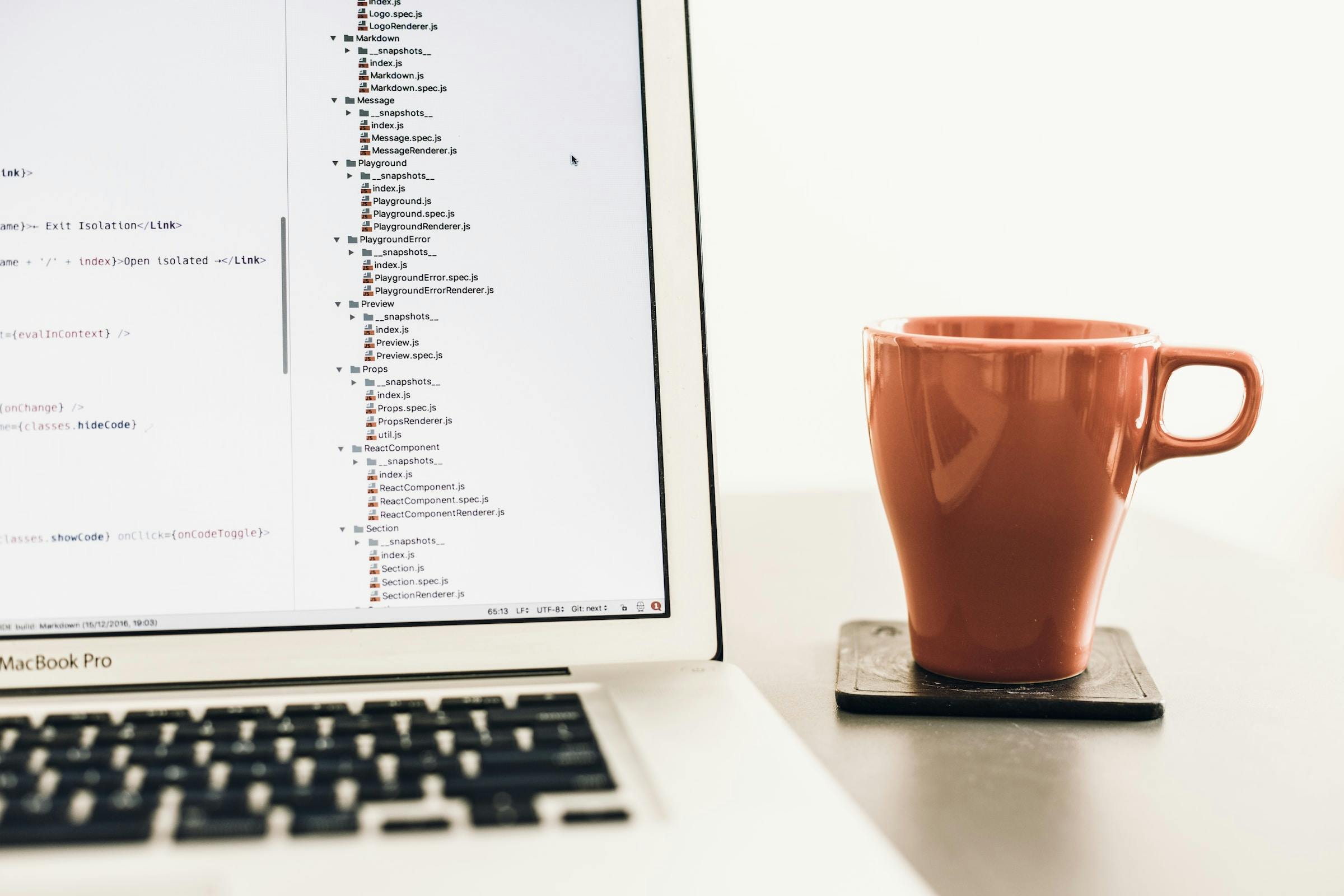
3. Why Use preventDefault()?
The primary reason to use preventDefault()
is to gain control over the behavior of web page elements and events. By preventing the default action, you can implement custom logic that suits the needs of your web application. Some everyday use cases include:
- Client-Side Form Validation: You can prevent form submission until user input meets specific criteria, providing users immediate feedback without reloading the page.
- Single Page Applications (SPAs): In SPAs, you often want to dynamically change the page's content without causing a full page refresh.
preventDefault()
helps achieve this by intercepting navigation events. - Enhancing User Experience: You can use
preventDefault()
to create interactive features like modal dialogs, tooltips, and custom dropdown menus.
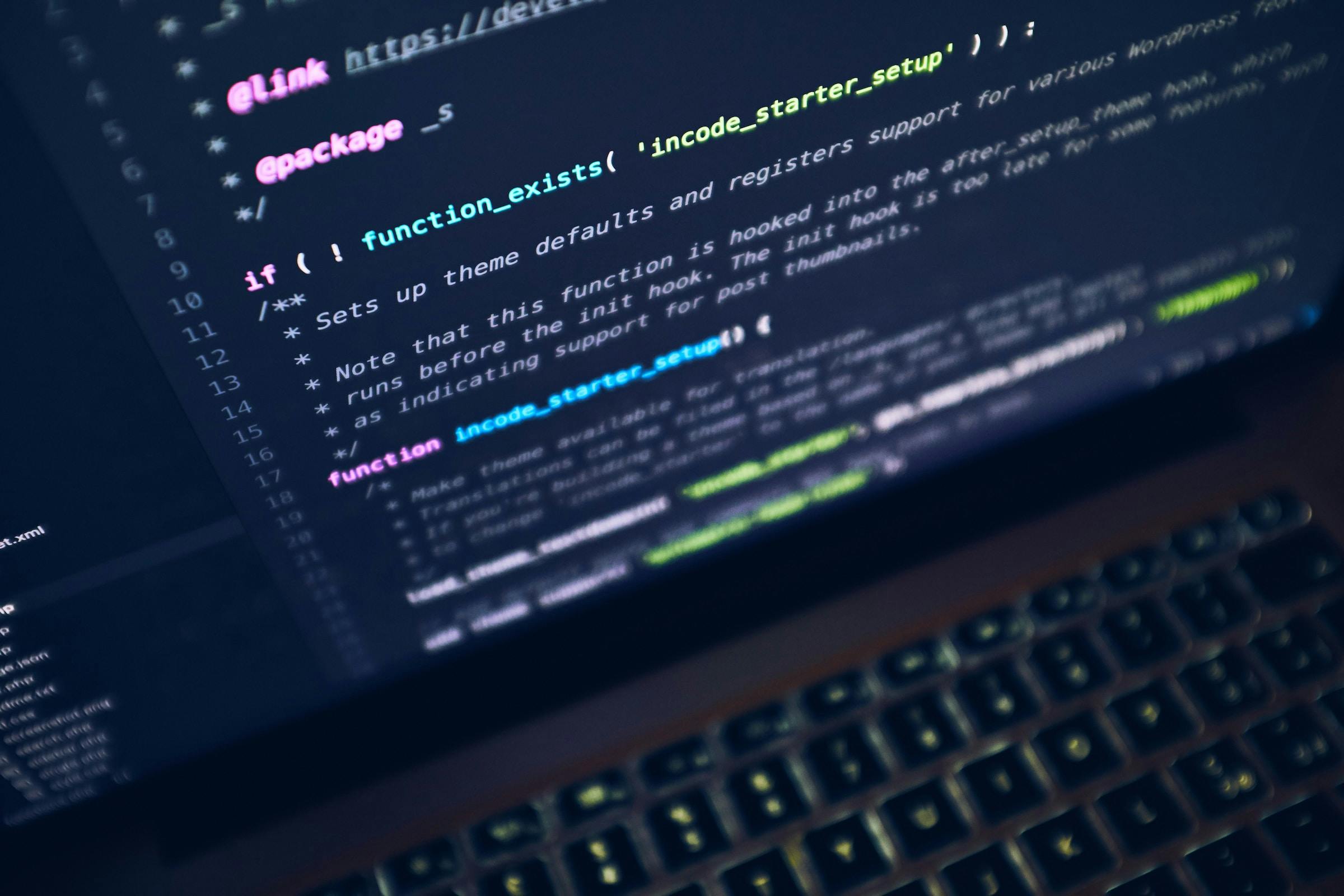
4. When to Use preventDefault()
While preventDefault() is a powerful tool, it should be used judiciously. It's essential to consider the user experience and accessibility when implementing custom event handling. Here are some guidelines for when to use preventDefault()
:
- Enhancing Usability: Use
preventDefault()
to improve the user experience, but ensure your custom behavior is intuitive and accessible. - Validating Input: Use it to validate user input on forms before submission, providing feedback without reloading the page.
- Single Page Applications: In SPAs, use
preventDefault()
to manage navigation and content loading without refreshing the entire page. - Keyboard Shortcuts: Use it to capture keyboard shortcuts, but make sure these shortcuts are documented and don't conflict with browser or assistive technology shortcuts.
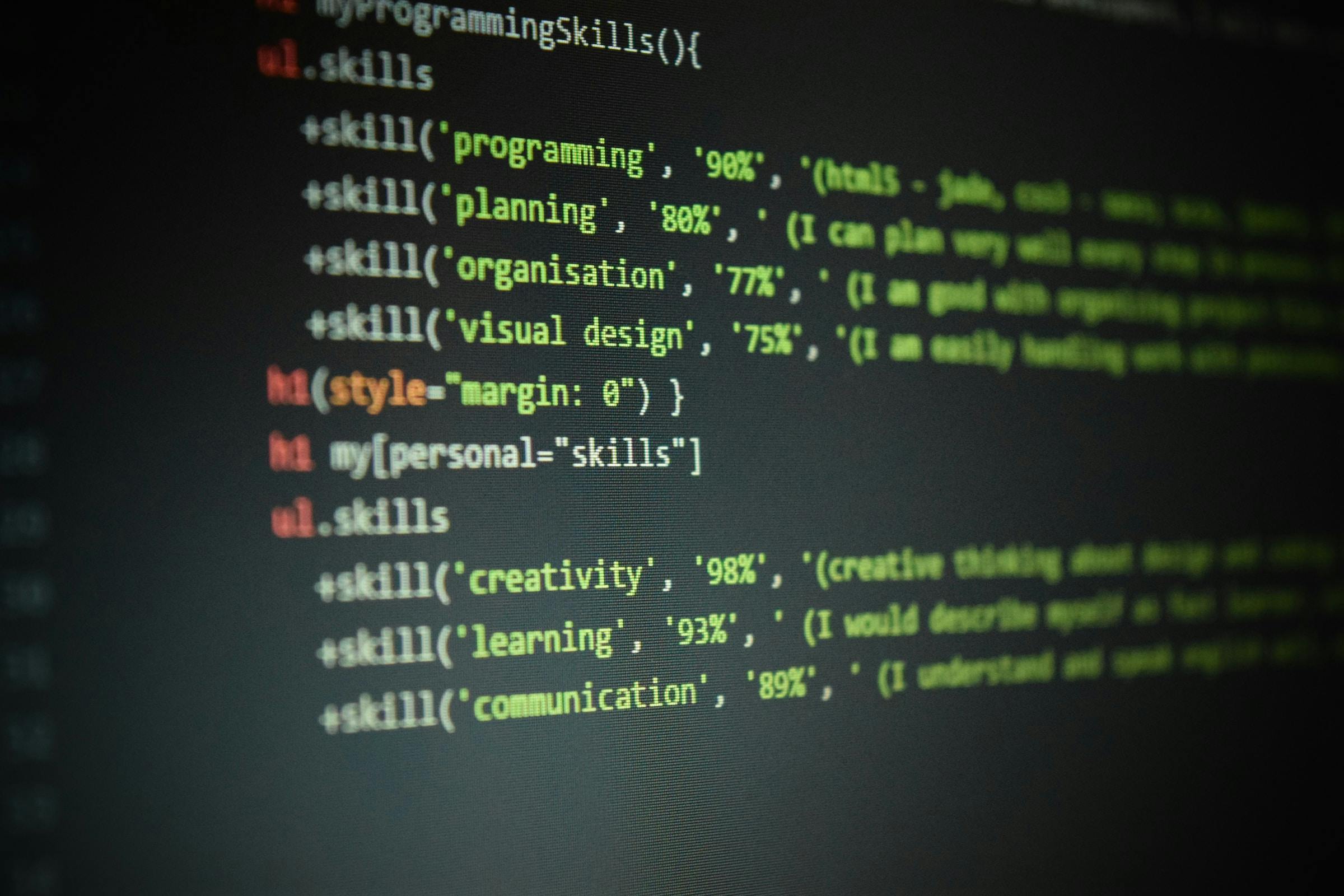
5. How to use it
// Preventing a Link from Navigating
document.querySelector('a').addEventListener('click', function (event) {
event.preventDefault();
// Custom logic here
});
// Preventing Form Submission
document.querySelector('form').addEventListener('submit', function (event) {
event.preventDefault();
// Custom form validation and submission logic here
});
// Capturing Key Presses
document.addEventListener('keydown', function (event) {
if (event.key === 'Enter') {
event.preventDefault();
// Custom action for Enter key press
}
});
In conclusion, the preventDefault()
function in JavaScript is a valuable tool for controlling and customizing the behavior of web page events. It allows developers to create more interactive and user-friendly web applications by preventing default actions and implementing custom logic. However, it should be used thoughtfully to ensure a positive user experience and accessibility. By understanding when and why to use preventDefault()
, you can take full advantage of its capabilities in your web development projects.